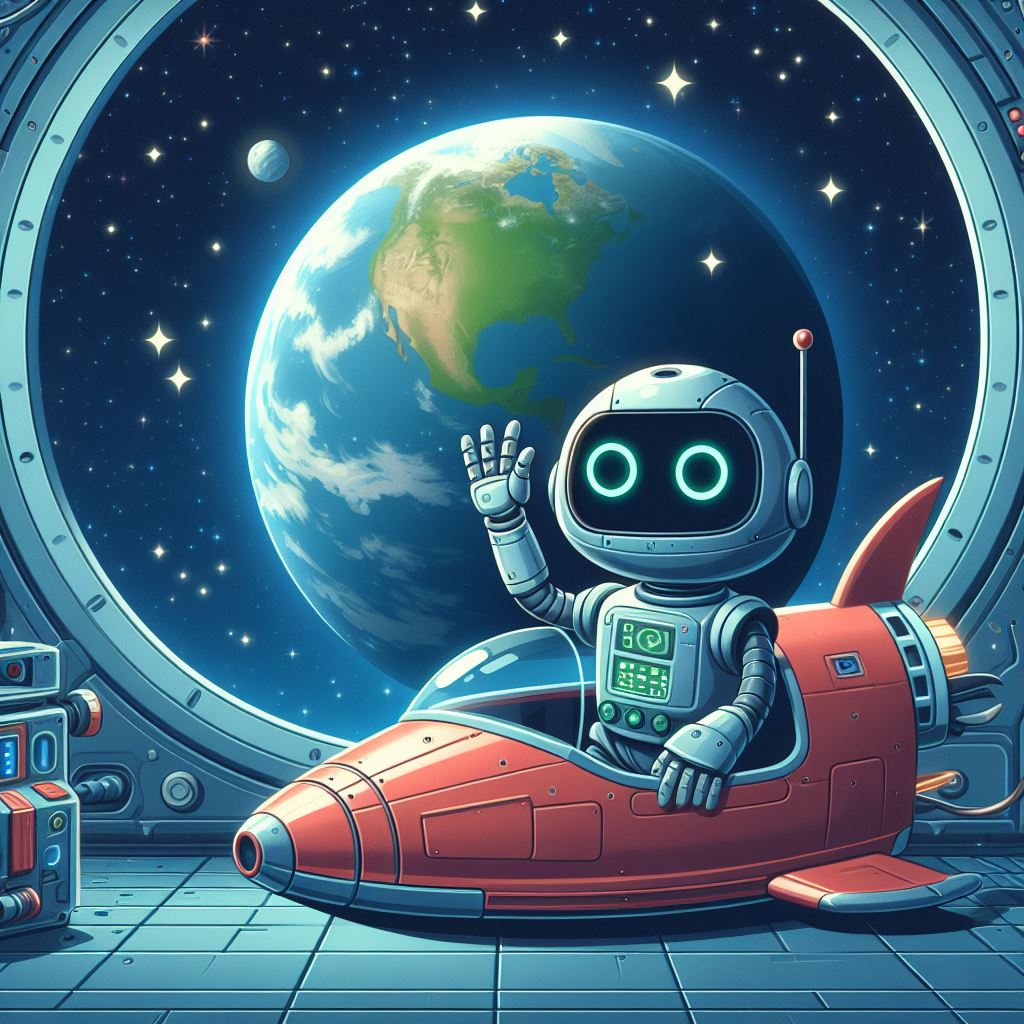
Content credentials: Generated with AI ∙ February 1, 2024 at 7:21 PM *
"Hello, World" in HTML
Published 2/1/2024
Difficulty Level:
Read time:
Hello, World.
As a rite of passage, when a student first learns how to write code in any language, the first lesson they are ever taught is known as the "Hello, World" program. The goal of this simple application is to get the student comfortable with using the programming language, and to show the basics of outputting a string of text to the display.
This tradition of learning to write a "Hello, World" program can be traced all the way back to one of the first authoritative programming books ever published: The C Programming Language, by Brian Kernighan and Dennis Ritchie. 1
HTML is the programming language that the Internet runs on. This website you are now reading was coded in HTML. Fortunately, for aspiring HTML coders, it is very easy to write a program in HTML. All you need is a computer and a plain text editor, such as Notepad in Windows, TextEdit in macOS, nano, or better yet - Vim, in Linux. Note: nano and Vim can also be used in macOS and in some versions of Windows.
You could, of course, use any text editor you'd like, such as the free and extremely popular Notepad++, Visual Studio Code, or even a paid App such as Sublime Text. Just make sure your files are saved with the extension ".html" and they will work in any modern web browser.
Because HTML files are stored as plain text, this makes things very easy for us. Take a look at the following code:
<!DOCTYPE html> <html lang="en"> <head> <title>"Hello, World." in HTML</title> </head> <body> <p>"Hello, World!"</p> </body> </html>
The code above will display the words "Hello, World!" in a browser. In fact, this code will be rendered by your browser exactly like this. Granted, it's not very much to look at, but it serves to illustrate how HTML code works. In order to understand this, we must understand HTML syntax and how HTML pages are structured into sections called "codeblocks". We must also understand the concept of HTML tags. Let's go through our code line by line, and by the end of this article you will know the basics of how to write code in HTML!
HTML Codeblocks and Tags
As previously stated, HTML is structured into "blocks" of code. In our little "Hello, World!" program above, there are five blocks. The very first line is technically not a block, it is a statement which tells the browser what type of document this is. Let's look at this a bit closer:
<!DOCTYPE html>
The
The rest of our code has five "codeblocks", which you will recognize because each block is encapsulated within a series of HTML "tags". These "tags" use special keywords that in HTML signify the start and end of a codeblock. The five blocks that we used in our code above are as follows:
<html> <head> <title> </title> </head> <body> <p> </p> </body> </html> Codeblocks: 1. <html> </html> 2. <head> </head> 3. <title> </title> 4. <body> </body> 5. <p> </p>
You will note that each codeblock starts and ends with the same tag, the only difference is that the ending tag has a forward slash "/" after the left angle bracket. This is precisely how HTML is written, and how the browser knows where the codeblock starts and where it ends. In HTML, these "tags" are individually referred to as "elements". They are the nuts and bolts of HTML pages which allow the Internet to function correctly.
Now you may be wondering if it matters whether or not HTML code is written in UPPERCASE, lowercase, or Title Case. The answer is that it depends. The text that appears within the <p> tags will be rendered on the display, and so you should type it the way you want the text to appear on screen. However, in some cases it doesn't matter how you write tags, the browser is programmed such that if you type <P> (UPPERCASE), it knows that you meant <p> (lowercase). However, to avoid confusion with other coders who may also be reading our code, we usually just type all tags in "lowercase", with some exceptions.
For example, you will note that the
You may also note that some "codeblocks" are positioned between other codeblocks, and that these blocks are "indented". For example, the <title> block is embedded within the <head> tag, and the <p> block is embedded within the <body> tag.
Furthermore, note that all of the other blocks are contained within the <html> and </html> tags, such that the <html> codeblock acts as a sort of "parent" block for the rest. That is exactly our intent for writing HTML code this way, and if you learn to code in other programming languages, you will note that they, too, use a similar type of coding structure. Therefore, it is important to understand this "parent-child" relationship between HTML tags.
The very basics of HTML require you to know what the
In addition, you should know that the
A Sample Block of Paragraphs
If you are interested in HTML development, you should try to experiment with this basic code. By now you should understand how everything that is typed within the
<body> <p> For example, if you wanted to write something in one paragraph, you would type the text within <p> blocks of an html document, like this. I have highlighted HTML tags in red for convenience.</p> <p> Then, if you wanted to type a different paragraph, you would simply type another paragraph using another pair of <p> and </p> tags. By doing this, you could put down in writing as much information as you need.</p> <p> You could even type an entire book in plain text, and then simply "copy" your text into these html paragraph blocks, but you may find that the more you type in HTML, the easier it gets to type directly within the code itself. This concludes my sample html paragraphs.</p> </body>
In this sense, HTML is one of the easiest and simplest programming languages that a person can learn to use, and it is very powerful as well. It is a language that, if learned properly, can be immediately applied in the real world. Of course, you will need to learn more in order to make the text look how you want it to, and for that we will eventually be learning CSS ('Cascading Style Sheets'), which work hand-in-hand with HTML. Without a doubt, it is very much worth it to learn to code in HTML.
Likewise, there are other elements that you can use to extend the functionality of HTML, so many, in fact, that you can make your page look exactly how you want, and if that is not enough for you, there are other programming languages that we can learn to provide even greater functionality, such as JavaScript and Python, but that's for another day.
I realize that this is an extremely basic tutorial for learning to code in HTML, in the future I will be adding to this so that we continue to learn together. The sky is the limit, and my hope is that I can somehow convince some of you who read this that you too, can learn to code.
- "Hello, World!" Wikipedia Entry
- World Wide Web Consortium (W3C) Website
- Mozilla Developer Network HTML Documentation
- "A history of HTML", as published on the World Wide Web Consortium's website
- An introduction to text editors, by the University of York